Input/Output Operations
Note
A practical example of the vast majority of the operations in this page are included in a working example in our GitHub respository
Input
load
Tensor* t1 = Tensor::load("mytensor.bin");
// [
// [1.00 2.00 3.00]
// [4.00 5.00 6.00]
// [7.00 8.00 9.00]
// ]
Output
save
-
void Tensor::save(const string &filename, string format = "")
Save tensor to a file.
- Parameters
filename – Name of the file to save the tensor to.
format – Filetype. The accepted filetypes are the following:
Images: png, bmp, tga, jpg, jpeg, hdr.
Text: csv, tsv, txt
Other: bin
- Returns
void
// Create matrix
Tensor* t1 = Tensor::range(1, 9); t1->reshape_({3, 3});
// [
// [1.00 2.00 3.00]
// [4.00 5.00 6.00]
// [7.00 8.00 9.00]
// ]
t1->save("mytensor.bin");
t1->save("mytensor.txt");
t1->save("mytensor.csv");
// Create matrix
Tensor* t1 = Tensor::range(1, 3*100*100);
// Reshape to a 4D tensor and normalize to RGB (0-255)
t1->reshape_({1, 3, 100, 100});
t1->normalize_(0, 255);
t1->save("mytensor.jpg");
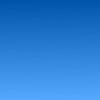